π§βπ» New post on TIL Computer: HTMX And POST Redirects
Just one of those days when I’m reminded that no matter how you think your users will use your system, there’s always someone that’ll push it to the breaking point. It’s also one of those days where I learnt that you can be a member of up to 113 groups in Active Directory.
Ok, this is strange. My work MacBook screen occasionally freezes when I interact with windows. It can’t be the screen as the mouse cursor moves just fine. But when I type or scroll inside a window, it just stops refreshing for a few seconds. Interestingly, the second display doesn’t have this issue.
Clip sharing is now available in Pocketcasts for Android! I was looking forward to this feature since I heard it was coming (this was why I was poking around About screens). Here’s my inaugural clip: a segment of Sharp Tech that I absolutely agree with. ποΈ
Weekly Update - 22 Sept 2024
No preface today. Let’s move on to the update.
Cyber Burger
Cyber Burger now has sound!
I started added some basic sound effects to the laser and the items flying across the screen.Β They may change, depending on how I find them after a while, but it’s a start. I do like how Pico-8 makes these easy to make: select a waveform, then just draw out the pitch and volume graphically:
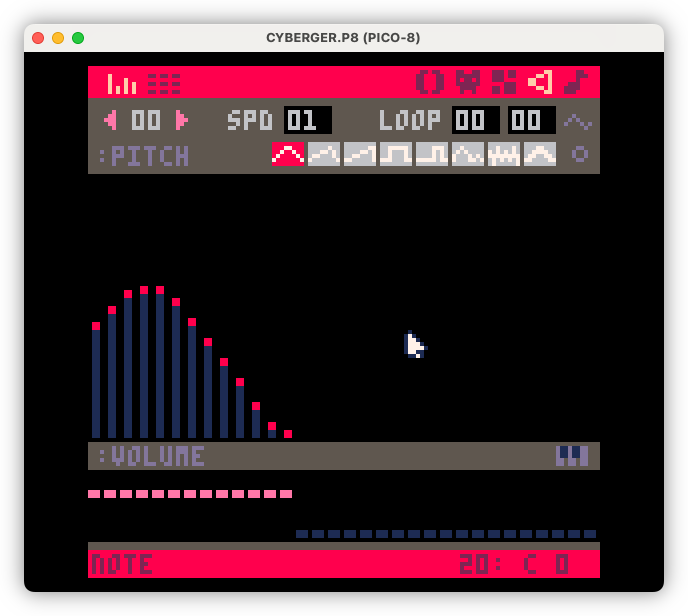
I also improved how items are spawned. Instead of the item types being completely random, they’re now taken from a list, which gets refilled and shuffled once it’s empty. This smoothes out the chance of seeing a particular item β no more waiting around for a bun bottoms to start your burger β while still making things random enough to be fun. The item types are distributed evenly, so every type will show up once per cycle. Maybe I’ll change this, but probably not, as it seems to be working reasonably well at the moment.
The Y location is now quantised a little so that items don’t completely overlap each other by a pixel or two. They can still be located on exactly the same Y position whichβ¦ is not great β I may need to take a look at that. But the slice spacing between each item looks good. Also, items now spawn in from the left as well as the right.
Lastly, I changed how demerits work. The previous version was not adding any when the player made a mistake. This made them pretty useless, and the game felt quite easy. So now, whenever the player catches an item in the basket that they weren’t suppose to, they get a demerit. If their burger gets too large, then get 2 demerits. To balance things out, the player now has 6 demerit points instead of 3, and every completed burger would remove one demerit until the player has zero again. I think this will require some more rebalancing, but it makes play a little more interesting.
Not sure what I’ll work on next. I’ll need to finish (or redo) the sounds, and then come up with some more tasks to do. I’m thinking either working on the difficulty curve, making things harder as the game progresses; adding power-ups and power-downs; or working on the start screen and menu. More on either of these in the future.
Blogging Tool
For my “extracurricular” activity this week, I spend some more time on Blogging Tool. I added the notion of jobs, which are essentially background tasks for long running processes. These can be monitored from within the Jobs section, which is accessible from the main nav:
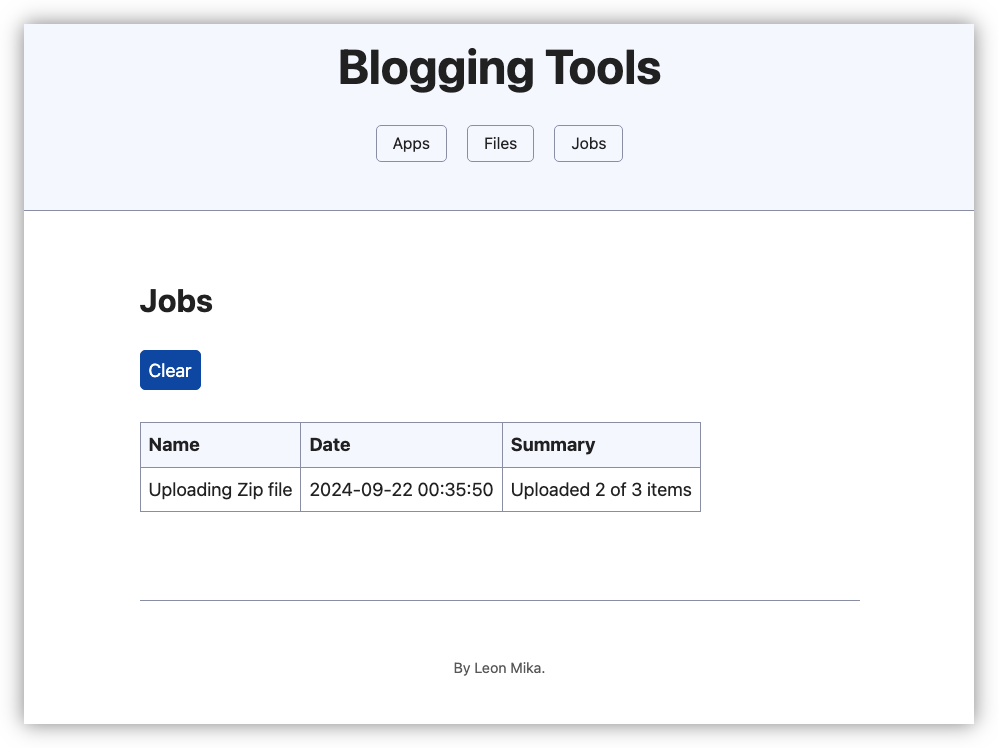
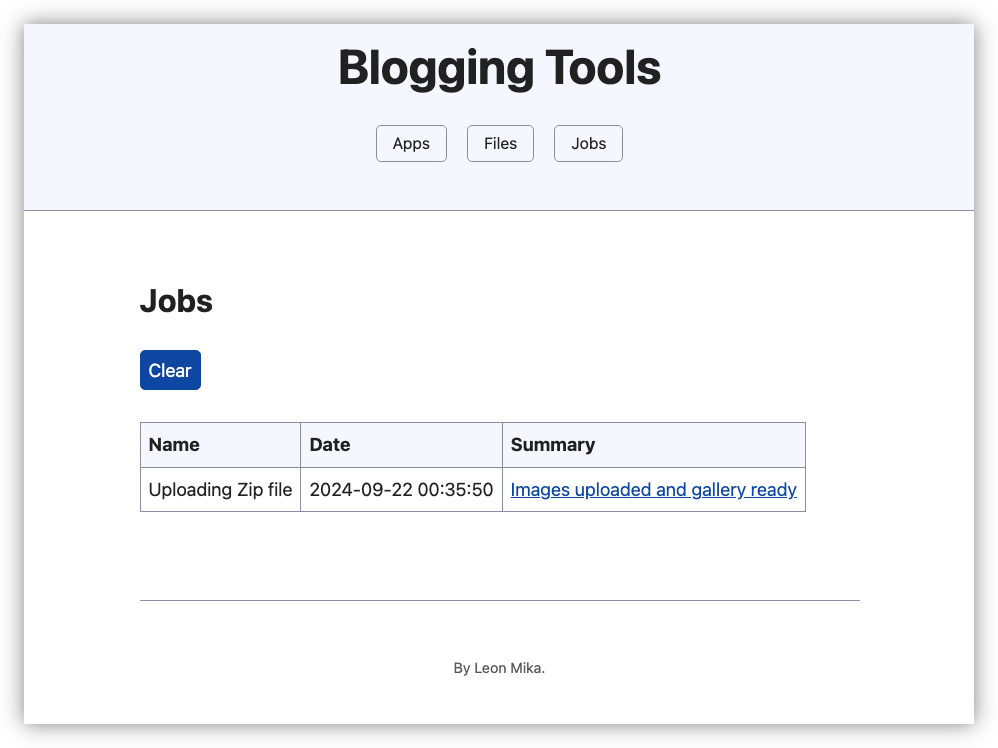
I’m recording jobs in the Sqlite3 database, including the ones that are currently running. Jobs have the ability to report progress by updating the “summary” message, which would update the job record in the database. I’m generally not too keen on having the database act as storage for something that could be updated quite frequently, but the alternative was keeping this progress information in memory or deploying something like Redis, both I felt were worse. Besides, I’m curious as to how well Sqlite3 handles status updates from a running job like this. I’m not expecting jobs to be updating there status too rapidly anyway, so it should be fine.
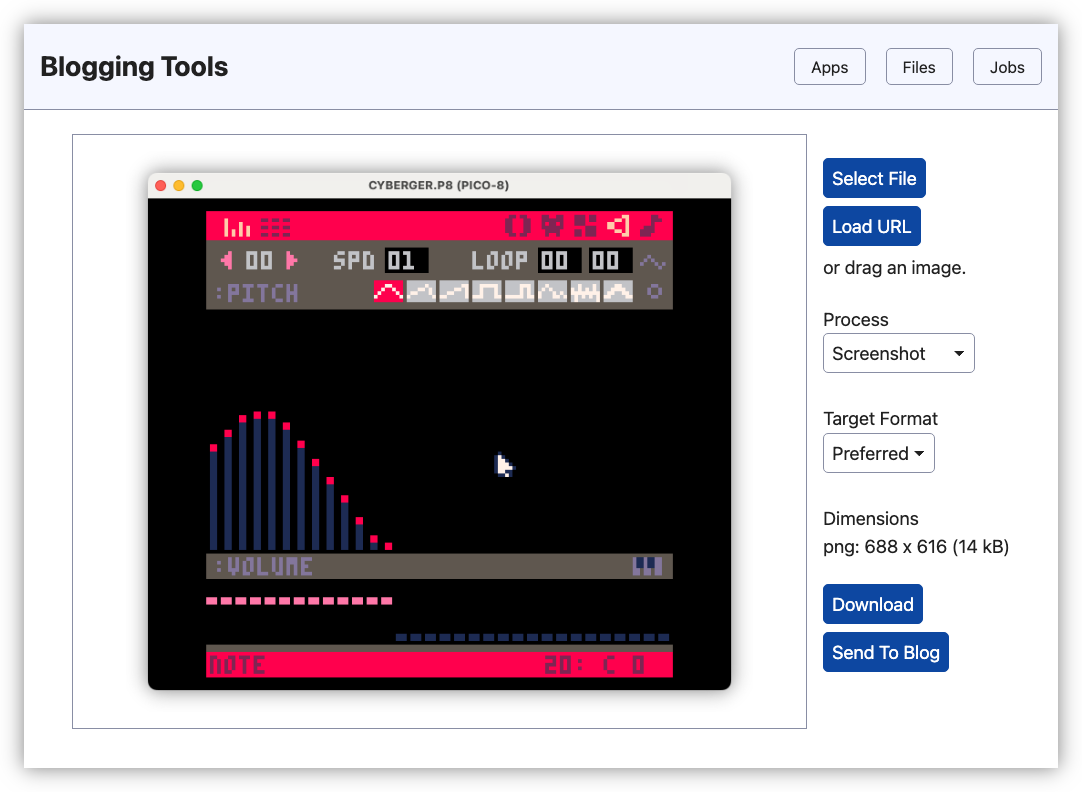
The Image App got a few changes as well. It’s now possible to import an image from a URL (this is done within the browser so is subject to all the cross-origin rules that entails). I added this just so that I can touch up my own images that I already uploaded to Micro.blog, without having to download the image first. Speaking of, there’s now an option to send a processed image to a blog directly from the app, saving yet another download step. I’ve got this configured to Micro.blog but it uses Micropub to do this so it could, in theory, be any blogging system that supports that API.
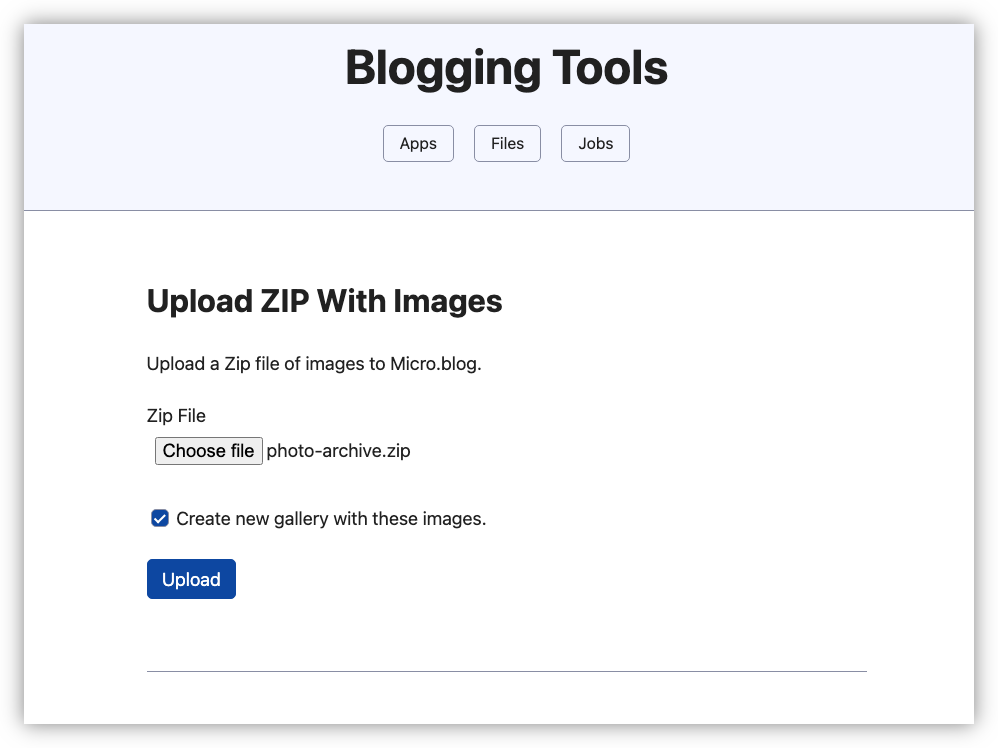
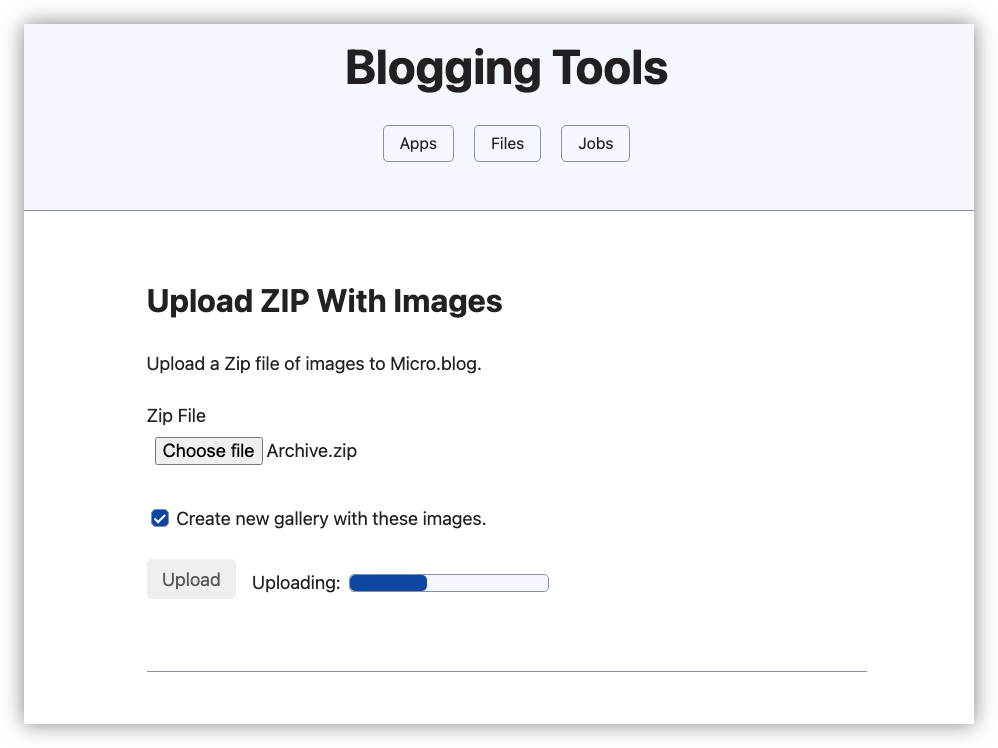
The last thing that was added is the ability to upload a Zip file of images to a Micropub endpoint, and start creating a gallery. This has been a feature that I wanted for a while. It always took me ages to prepare a photo gallery in Micro.blog. I know there are apps which help here, but they’re for iOS and just don’t fit my workflow. Now, whenever I want to add a photo gallery, I can select the images from Google photos, download them as a Zip file, then directly upload them to Micro.blog in one hit. The upload can take as long as it needs to (this uses the new jobs system as well), and once they’re done, Blogging Tool will create a gallery for me so I can write the captions. To be able to do this without clicking around as much as I did is rather exciting.
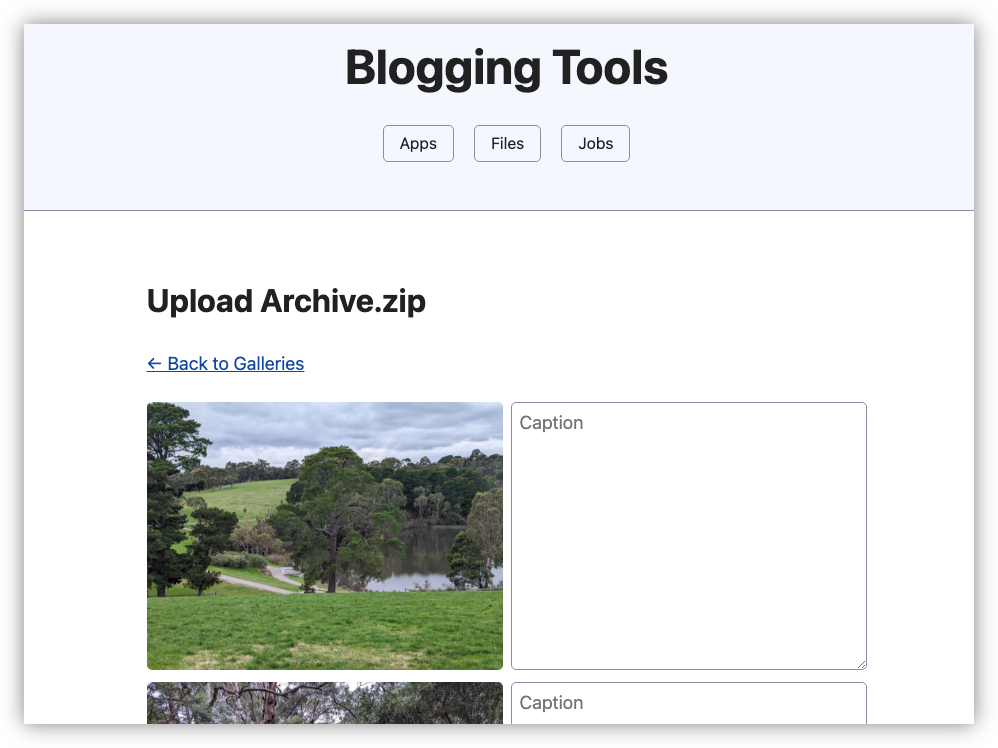
Or at least it will be, when I find out how I can get large uploads working. Uploading a 27 MB Zip file in development was not an issue, but when I deployed it to my Dokku server and try it for real, I kept getting 502 errors. I’m not sure what’s causing these yet: both Nginx and Blogging Tool have been configured to accept files much larger than this, and there are no logs in either to suggest a problem. There might be a timeout somewhere that needs to be raised. In any case, hopefully this is the last hurdle I need to clear to get this working. Anyway, that’s all for this week. Oh, actually, one more thing: while working on the UI for the Upload Zip screen, I found that I never got around to changing the instructions on the Transcode Audio screen after copying and pasting the HTML from the New Gallery screen:
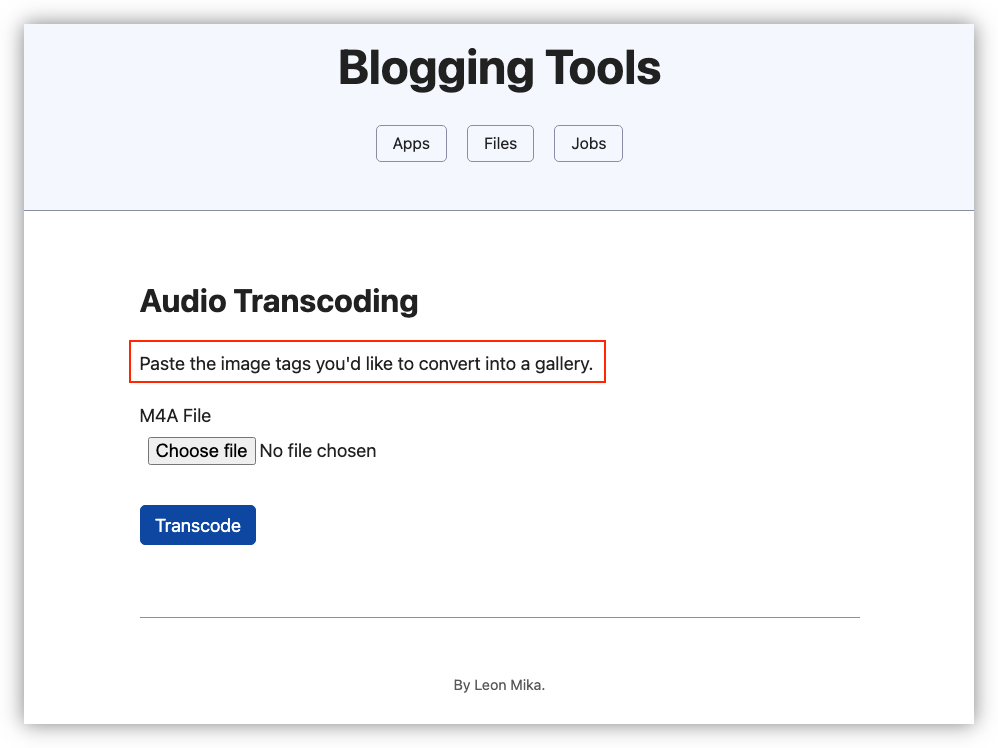
Just goes to show how little I pay attention to this copy, even while working on my own apps. π
And that swoop-o-meter just keeps going up. We’re up to 4 noisy miners now. π·ββοΈ
Every problem at every company Iβve ever worked at eventually boils down to βplease dear god can we just hire people who know how to write HTML and CSS.β
I know bugger all about the world of front-end web development. But seeing how quickly it takes me to get changes made and deployed using just these technologies, verses dealing with the mountain of JavaScript for an SPA, leaves me convinced that those that embrace HTML and CSS have a significant advantage over those that don’t.
I couldn’t for the life of me find out how to use HTMX with import maps. Importing it into a JavaScript module seems to activate all the HTML attributes just fine, but I had no access to the htmx
global, not even through the window
object. Hope support for this is added soon.
Huh, that’s interesting. I just noticed that there’s an option to go to Automattic jobs page at the bottom of Pocketcast’s About screen. Nothing wrong with that. In fact, it may make for a good way to find developers. Who else would go to the About screen of mobile apps? π
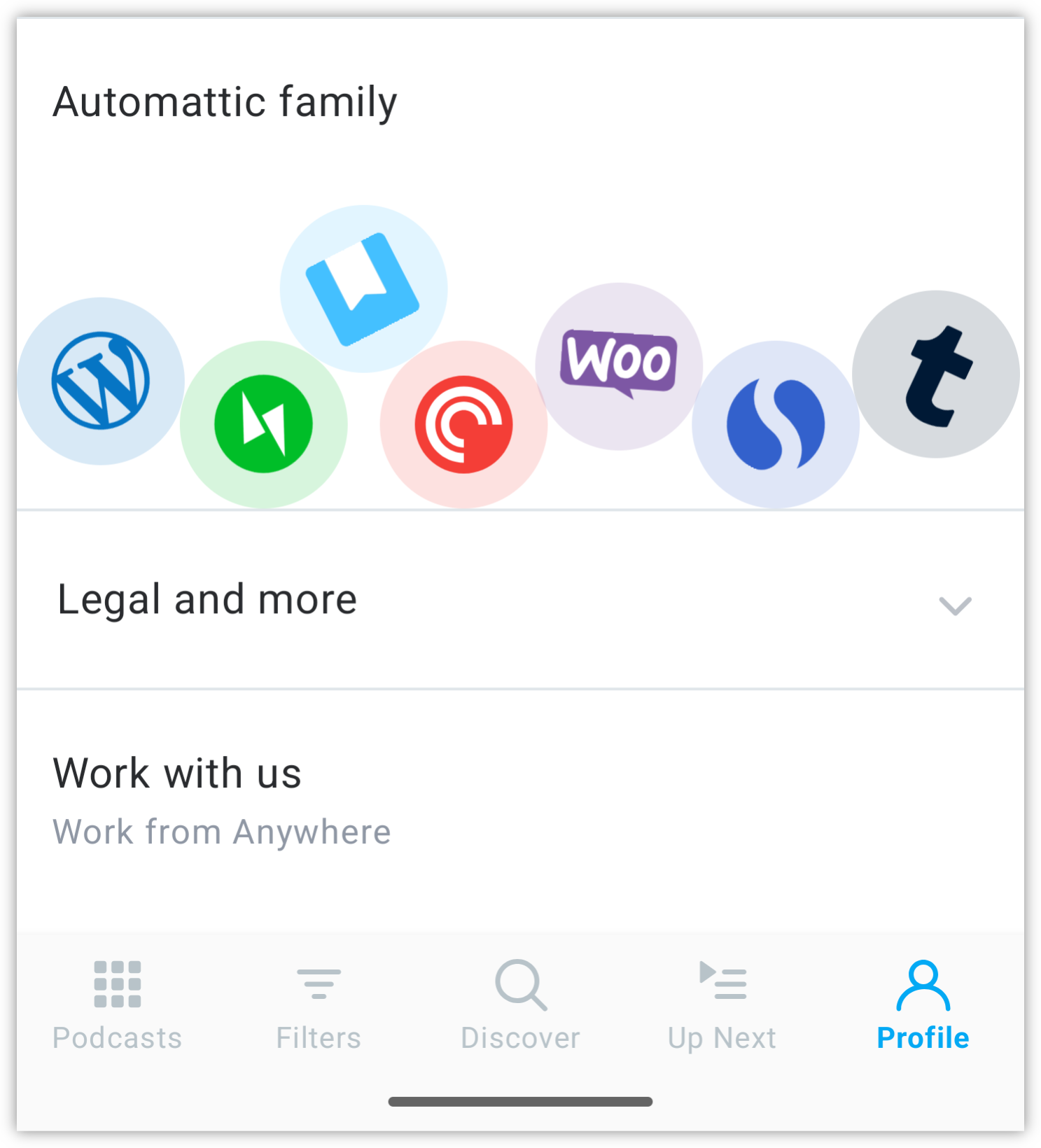
πΊ Dune: Part 2 (2024)
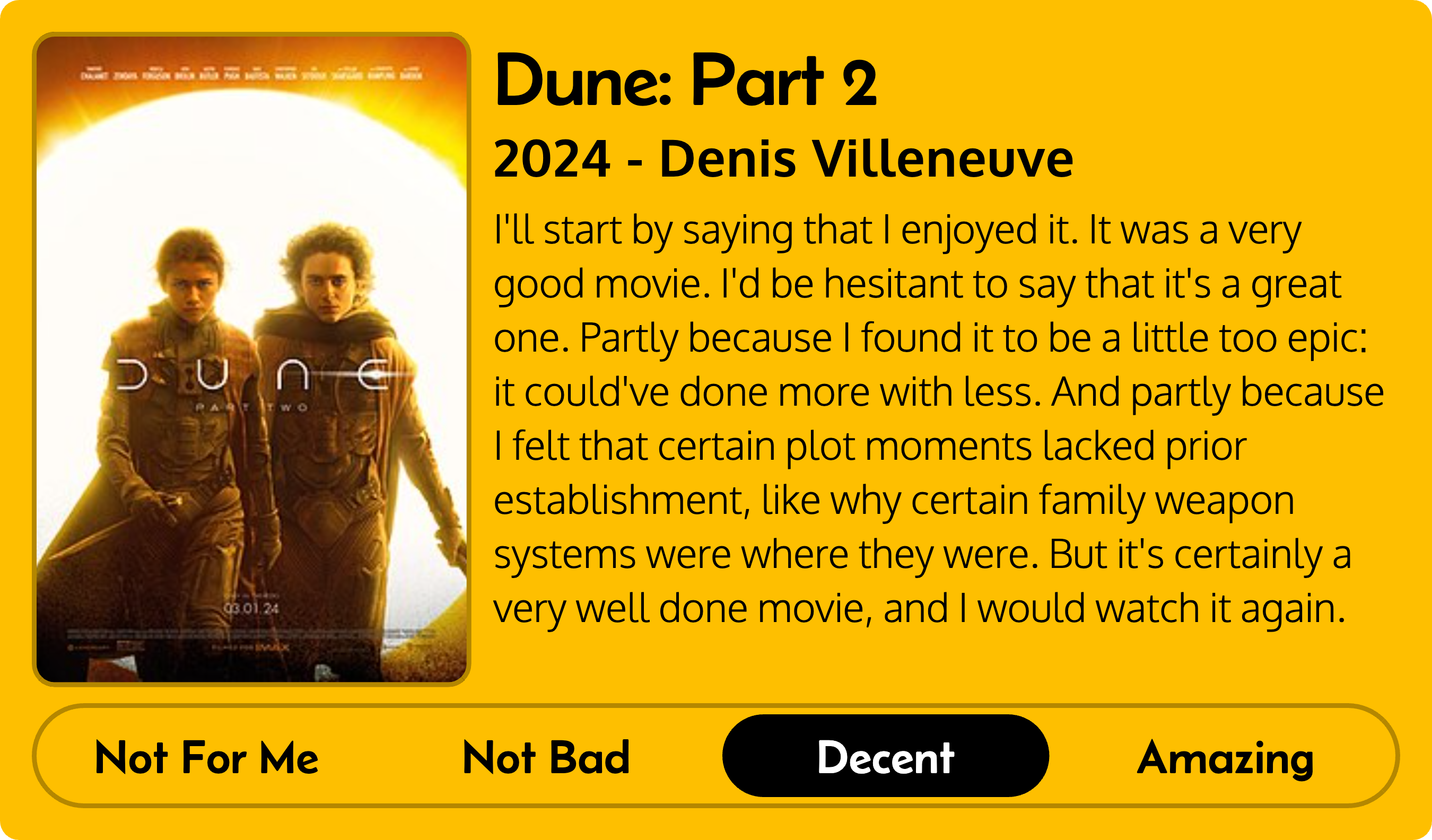
Micro-fiction: Get A Horse
Trying something new here. I came up with the concept of this short-story while riding home on the tram yesterday. The rest of it sort-of fell into place when I woke up at 5AM this morning, unable to get back to sleep. Hope you enjoy it.
Josh was riding the scooter on the city footpath, not trying super hard to avoid the other pedestrians. He was going at a speed that was both unsafe and illegal, but it was the only speed he knew that would prevent that horse from showing up. Besides, he had something that he needed to do, and it was only at such reckless speeds that he knew that that thing would work. Well, he didnβt know; but being at his wits’ end after trying everything else, he had to try this. He picked his target ahead and sped up towards it. Good thing he was wearing his helmet.
Josh never used these sorts of scooters before the collision two weeks ago. He was walking to work that day, when he saw someone on such a scooter coming towards him, helmet on head. The rider was going a ridiculous speed, and Josh tried to get out of his way as he approached, but the scooter driver turned towards him, not slowing down at all. Josh tried again but was not fast enough. The scooter rider ran straight into him and bowled him over onto the footpath. Before Josh could gather himself, the scooter rider slap his helmet onto Josh’s head and shouted, βGet a horse!β He got back onto the scooter and sped away.
Josh got up, fighting the various aching muscles from the fall. He dusted himself down, took the helmet from his head and looked at it. It was very uncharacteristic of those worn by scooter riders. Most of them were plastic things, either green or orange, yet this one was grey, made of solid leather that was slightly fuzzy to the touch. Josh looked inside the rim and found some printed writing: Wilkinsons Equestrian Helmet. One side fits all. The one was underlined with some black marker.
Josh put the helmet in his backpack and was about to resume his commute, when he stopped in place. Several metres away, a white horse stood, staring at him. Or at least it looked like a horse. The vision was misty and slightly transparent, giving the sense that it was not real. Yet after blinking and clearing his eyes, it didnβt go away. Josh started to move towards it, and when he was just within arms reach, it disappeared. Josh shook his head, and starting walking. But when he turned the next corner, there it was again: a horse, standing in the middle of the footpath several metres away, staring at him intently.
Since that day that horse has been haunting Josh. On his walk, at his workplace, in his home, even on the tram. Always staring, always outside of reach. Either standing in his path or following close behind him. The vision will go whenever Josh approached it, only to reappear when he turned to look in another direction. Naturally, no one else could see it. When that horse was in a public place, people seemed to instinctively walk around it. Yet when he asked them if they could see it, they had no idea what he was talking about. But Josh couldnβt do anything to stop seeing it. At every waking hour of the day, from when he got out of bed to when he got back in, there it was, always staring. Never looking away.
And he knew it had something to do with that helmet. He tried a few things to dispel the vision, such as leaving the helmet at home or trying to give to random strangers (who always refused it). Yet nothing worked to clear the vision. That is, nothing other than what had worked on him. Now was the time to test that theory out.
His target was ahead, a man in a business suit walking at a leisurely pace. He had his back to Josh, so he couldn’t see Josh turn his scooter towards him and accelerate. The gap between them rapidly closed, and Josh made contact with the man, slowing a little to avoid significant injury, but still fast enough to knock him over. Josh got off the scooter and stood by the man, sprawled on the footpath. Once again the horse appeared, as he knew it would. He looked down to see the man starting to get up. Josh had to go for it now! He took his helmet from his head, slapped it on the man and shouted, βGet a horse!β
Josh got back on the scooter and sped away for few seconds, then stopped to look behind him. He saw the man back on his feet, helmet in hand, looking at it much like Josh did a fortnight ago. He saw the horse as well, but this time it had its back to Josh, staring intently at the man, yet Josh could see that the man hasn’t noticed yet. He could see the man put the helmet by side of the road and walk away, turning a corner. The horse was fading from Josh’s eyes, yet it was still visible enough for Josh to see it follow the man around the corner, several metres behind.
More Hemispheric Views #120 feedback: not that it happens often, but I too find it strange to be addressed as “sir”. I mean, I do appreciate the respect it imbues, but it adds an air of distance that I find slightly off-putting. Much rather be addressed as “mate.”
The whole segment on incorrect names on Hemispheric Views #120 was very enjoyable but this story from Scotty J. was the capper. I burst into laughter when I heard it. ποΈ
[Source not online
at time of site build.]
Oof, how to feel old: I’ve done everything on this list, except for number 9, and only if you take the strict definition of “boombox” (I’ve listen to music on a radio with a cassette deck, for example). Oh, and I haven’t done number 19 either, but that’s because my parents had no interested in buying one. But I did have access to a encyclopaedia, so I think that’s close enough. Interestingly, the only time I ever sent a fax was in 2016.
There’s an old iMac in our office that has the dictionary screen-saver. You know the one with the blue background with words scrolling across the screen, then one pauses and the definition appears? Such a good screen-saver. Wish I had a reason to turn it on on my machines.
Slack has got a lot of work to do on their lists feature. It’s just feels horrible to use at the moment. It takes three clicks to select a cell, scroll events don’t work, and numerical fields are misaligned in edit mode. I can see this feature being useful. It just needs way more time in the oven.
There’s no such thing as a finite number of niches. People are doing, watching, and supporting interests that would’ve never crossed your mind. The internet is a really large place.
This epiphany came to me today as we were discussing, of all things, TikTok videos. I don’t use TikTok myself, but I work with people who do β or at least are more clued in to what goes on over there than I am1 β and it was quite eye-opening to hear what people would support.
-
Generally not a hard thing to do. ↩︎
What I would give to be riding on a Hitachi, with pull down windows, so that I can yell at some kids who thought trespassing on the railway line is a smart idea. π
It’s also just a fun train to ride in, especially in summer (I’m not kidding about this last point π).
Forgotten how long it takes for AMIs to bake using Amazon Image Builder (up to 40 minutes). β³